게으른개발너D
[JS] Momentum App 2 - Clock 본문
Intervals and Timeouts
1. interval
시간에따라 무언가 표현하려할 때 interval과 timeout을 사용할 수 있다.
interval은 특정한 시간마다 특정한 이벤트를 실행시킬 수 있는 것이다.
예를 들어 2초마다 주식 시장을 확인하던가 1초마다 코인 업다운을 확인하던가 하는 것들이다.
function sayHello() {
console.log("hello");
}
setInterval(sayHello, 5000);
setInterval(실행시킬 이벤트, 반복할 시간)
위의 코드는 5초마다 콘솔창에 hello를 찍어낸다.
2. timeout
timeout은 지정한 시간이 지난 후 어떠한 이벤트를 실행시킬 수 있는 것이다.
function sayHello() {
console.log("hello");
}
setTimeout(sayHello, 5000);
setTimeout(실행시킬 이벤트, 타이머 시간);
위의 코드는 5초 후에 콘솔창에 hello를 한번 찍어낸다.
Dates
Javascript에서는 Date로 날짜와 시간을 표시할 수 있다.
console.dir(Date)를 실행 후 prototype을 보면 그 안에 있는 다양한 기능을 볼 수 있다.
new Date()를 하면 현재 날짜와 시간을 표시할 수 있다.
const clock = document.querySelect("h2#clock");
function getClock(){
const date = new Date();
clock.innerText = `${date.getHours()}:${date.getMinutes()}:${date.getSeconds()}`;
}
h2 태그에 현재 시간을 표시하는 함수를 만들었다.
초단위로 시간이 변하는 것을 보여주려면 interval을 쓰면 된다.
setInterval(getClock, 1000);
1초마다 getClock함수를 호출하여 초단위로 시간이 변하는 걸 화면에서 볼 수 있다.

하지만 이렇게 하면 1초 후에 getClock 함수가 호출되므로 맨 처음 화면에선 시간을 볼 수 없게 된다.
그래서 화면에 진입하자마자 getClock 함수를 한번 호출한다.
getClock();
setInterval(getClock, 1000);
PadStart
시계를 그냥 위의 코드처럼 구현하면 1시 18분 5초는 1:18:5 이렇게 나타난다.
정말.. 쫌... 구리다...
그걸 해결하기 위해 한자리 숫자는 앞에 0을 붙여야한다.
지금까지는 그걸 구현하기 위해 if, else를 이용했었는데 padStart라는 캡짱인걸 이용하면 된다. 진심 충격임...
padStart는 padding을 얼마큼 줄건데 그게 모지라면 앞부분을 이렇게 시작하겠다라는 의미이다.
그래서 padEnd도 있다.
"1".padStart(2, "0");
"string".padStart(원하는 string length, "채워줄 string");
1은 2글자가 아니므로 앞에 0이 붙어서 01이 프린트 된다.
앞에서 구현한 현재 시간을 padStart로 이쁘게 나타내보자.
function getClock() {
const date = new Date();
const hours = date.getHours().padStart(2, "0");
const minutes = date.getMinutes().padStart(2, "0");
const seconds = date.getSeconds().padStart(2, "0");
clock.innerText = `${hours}:${minutes}:${seconds}`;
}
이렇게 구현하면 맞을까? 땡!!
date.getHours()는 number이기 때문에 에러가 난다.
padStart는 string에 쓸 수 있는 기능이다.
따라서 시간을 string으로 변환시켜줘야한다.
integer을 string으로 변환하는 방법은 integer을 String으로 감싸주기만 하면 된다. 이렇게 편할 수가!!
function getClock() {
const date = new Date();
const hours = String(date.getHours()).padStart(2, "0");
const minutes = String(date.getMinutes()).padStart(2, "0");
const seconds = String(date.getSeconds()).padStart(2, "0");
clock.innerText = `${hours}:${minutes}:${seconds}`;
}
이렇게 String으로 감싸준 시간이 한글자면 padStart를 이용하여 앞에 0이 붙도록 만들어 줬다.
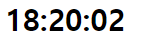
clock.js
const clock = document.querySelector("h2#clock");
function getClock() {
const date = new Date();
const hours = String(date.getHours()).padStart(2, "0");
const minutes = String(date.getMinutes()).padStart(2, "0");
const seconds = String(date.getSeconds()).padStart(2, "0");
clock.innerText = `${hours}:${minutes}:${seconds}`;
}
getClock();
setInterval(getClock, 1000);
'프로젝트 > Side Project' 카테고리의 다른 글
[JS] Momentum App 6 - Style (0) | 2023.02.13 |
---|---|
[JS] Momentum App 5 - Weather (0) | 2023.02.13 |
[JS] Momentum App 4 - To Do (0) | 2023.02.13 |
[JS] Momentum App 3 - Quotes and Background (0) | 2023.02.13 |
[JS] Momentum App 1 - Login (0) | 2023.02.12 |