게으른개발너D
[JS] Momentum App 3 - Quotes and Background 본문
Quotes
명언을 랜덤으로 보여주기위해 일단 명언 11가지를 Array와 Object로 구현했다.
const quotes = [
{
quote: "Be yourself; everyone else is already taken.",
author: "Oscar Wilde"
},
{
quote: "A room without books is like a body without a soul.",
author: "Marcus Tullius Cicero"
},
{
quote: "Without music, life would be a mistake.",
author: "Friedrich Nietzsche"
},
{
quote: "You know you're in love when you can't fall asleep because reality is finally better than your dreams.",
author: "Dr. Seuss"
},
{
quote: "You only live once, but if you do it right, once is enough.",
author: "Mae West"
},
{
quote: "In three words I can sum up everything I've learned about life: it goes on.",
author: "Robert Frost"
},
{
quote: "If you want to know what a man's like, take a good look at how he treats his inferiors, not his equals.",
author: "J.K. Rowling"
},
{
quote: "If you tell the truth, you don't have to remember anything.",
author: "Mark Twain"
},
{
quote: "People will never forget how you made them feel.",
author: "Maya Angelou"
},
{
quote: "Do it yourself!",
author: "Lazy Bone"
},
{
quote: "What't matter? Not my business.",
author: "Hwayeon Song"
}
];
이 열한가지 명언을 랜덤으로 화면에 보여주기 위해 Math를 이용한다.
Math.random()을 콘솔에 찍으면 0.XXXXXXX 라는 float 숫자를 랜덤으로 가져온다.

우리가 원하는건 0~10까지의 인덱스이기 때문에 Math.random()에 명언의 갯수인 11을 곱하면
0 초과, 11 미만의 숫자가 탄생한다.
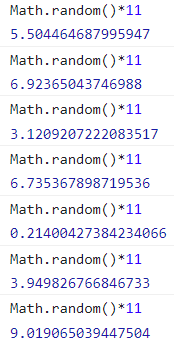
하지만 우리는 점 뒤에 붙은 숫자는 필요가 없다. 이때 필요한게 Math.floor()이다.
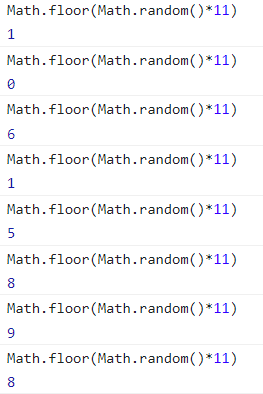
Math.floor()은 버림
Math.ceil()은 올림
Math.round()는 반올림
const todaysQuote = quotes[Math.floor(Math.random() * 11)];
이렇게 하면 인덱스 0~10 까지의 명언을 랜덤으로 불러오게 된다.
하지만 11이라는 숫자를 그대로 둬도 되겠는가?
만약 명언을 몇개 더 추가하게 되면 몇몇의 명언을 불러 올 수 없게 된다.
따라서 11 대신 Array의 갯수를 넣는다.
화면에 표시하게 위해 이렇게 작성하면 되겠다.
const quote = document.querySelector("#quote span:first-child");
const author = document.querySelector("#quote span:last-child");
const todaysQuote = quotes[Math.floor(Math.random() * quotes.length)];
quote.innerText = todaysQuote .quote;
author.innerText = todaysQuote .author;
Background
화면에 이미지를 표시하기 위해 img 폴더를 만들고 그 안에 이미지를 넣었다.
이미지를 랜덤으로 불러오기 위해 Array에 담았다.
const images = [
"0.jpg", "1.jpg", "2.jpg", "3.jpg", "4.jpg", "5.jpg", "6.jpg", "7.jpg"
, "8.jpg", "9.jpg", "10.jpg", "11.jpg", "12.jpg", "13.jpg", "14.jpg"
, "15.jpg", "16.jpg", "17.jpg", "18.jpg", "19.jpg", "20.jpg", "21.jpg"
, "22.jpg", "23.jpg", "24.jpg", "25.jpg", "26.jpg", "27.jpg", "28.jpg"
];
이미지를 랜덤으로 불러오는 방법은 위에서 했던 명언 랜덤 불러오기와 같다.
const chosenImage = images[Math.floor(Math.random() * images.length)];
HTML 파일에 이미지 태그를 넣을 수도 있지만 JavaScript로 이미지를 만들어보자.
const bgImage = document.createEliment("img");
bgImage.src = `img/${chosenImage}`;
document.createEliment()로 이미지 태그를 만들 수 있다.
그리고 그 안에 속성인 src로 랜덤으로 가져온 이미지 경로를 추가해 주었다.
const bgImage = document.createElement("img");
bgImage.src = `img/${chosenImage}`;
document.body.appendChild(bgImage);
마지막으로 body에 appendChild()를 해주면 body 태그 아래부분에 랜덤으로 선택된 이미지 태그가 들어간다.
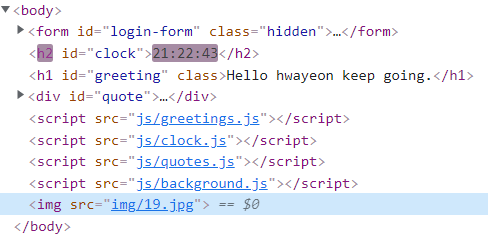
quotes.js
const quotes = [
{
quote: "Be yourself; everyone else is already taken.",
author: "Oscar Wilde"
},
{
quote: "A room without books is like a body without a soul.",
author: "Marcus Tullius Cicero"
},
...
];
const quote = document.querySelector("#quote span:first-child");
const author = document.querySelector("#quote span:last-child");
const todaysQuote = quotes[Math.floor(Math.random() * quotes.length)];
quote.innerText = todaysQuote.quote;
author.innerText = todaysQuote.author;
backgrouond.js
const images = [
"0.jpg", "1.jpg", "2.jpg", "3.jpg", "4.jpg", "5.jpg", "6.jpg",
...
];
const chosenImage = images[Math.floor(Math.random() * images.length)];
const bgImage = document.createElement("img");
bgImage.src = `img/${chosenImage}`;
document.body.appendChild(bgImage);
'프로젝트 > Side Project' 카테고리의 다른 글
[JS] Momentum App 6 - Style (0) | 2023.02.13 |
---|---|
[JS] Momentum App 5 - Weather (0) | 2023.02.13 |
[JS] Momentum App 4 - To Do (0) | 2023.02.13 |
[JS] Momentum App 2 - Clock (0) | 2023.02.12 |
[JS] Momentum App 1 - Login (0) | 2023.02.12 |